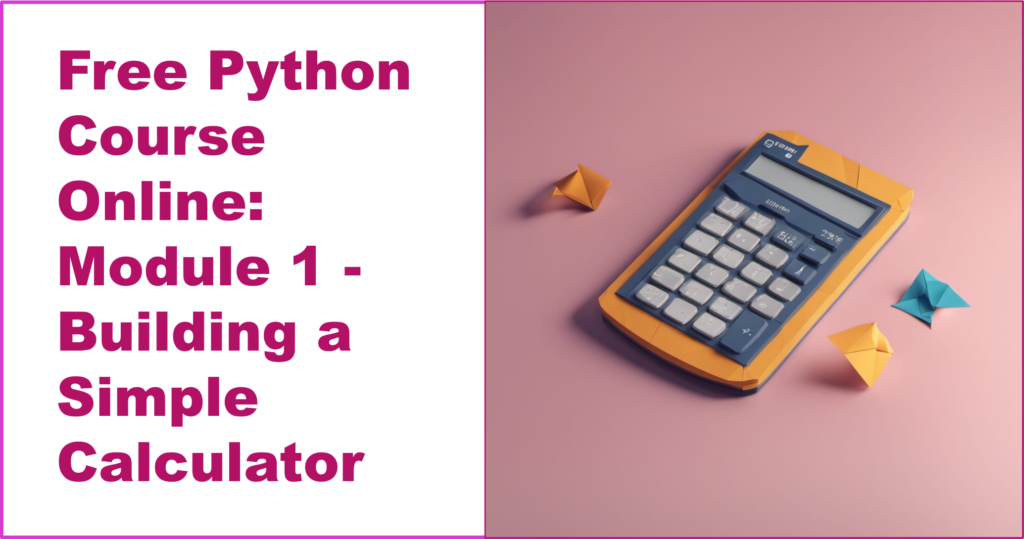
Crafting a Powerful Calculator from Scratch
Thank you for reading this post, don't forget to share! website average bounce rate Buy traffic for your website
Are you ready to embark on a journey into the world of Python programming? In this article, we’ll dive into the fundamentals of Python by creating a simple calculator from scratch. Whether you’re a coding novice or looking to refresh your skills, join us as we explore variables, data types, and basic operations, ultimately culminating in the creation of your very own calculator.
Understanding Python Basics
1. Introduction to Python Basics
Python, a versatile and powerful programming language, has gained immense popularity due to its readability and simplicity. It’s the go-to language for beginners and experts alike. To kickstart your Python journey, let’s begin by setting up your Python environment and writing your first code.
What is Python and why is it popular?
Python is an open-source, high-level programming language known for its easy-to-understand syntax. Its popularity stems from its versatility – it’s used for web development, data analysis, artificial intelligence, automation, and more. Python’s readability and extensive libraries make it a favorite among developers.
Setting up your Python environment
Before we start coding, you need to set up your Python environment. Visit Python’s official website to download the latest version for your operating system. Follow the installation instructions, and you’re ready to roll.
Writing your first Python code
Let’s dive into your first Python code. Open a text editor (like Notepad on Windows, or TextEdit on macOS) and type the following:
1 |
print("Hello, Python!") |
Save the file with a .py extension, such as first_program.py. Open your command-line interface, navigate to the file’s directory, and run the program by entering:
1 |
python first_program.py |
Expected Output:
1 |
Hello, Python! |
With this simple step, you’ve run your first Python program! In the next section, we’ll delve into variables and data types.
2. Understanding Variables and Data Types
In Python, variables are like containers that hold different types of data. Let’s explore the basic data types and learn how to declare variables.
Exploring different data types: int, float, string
Python supports various data types, including integers (int), floating-point numbers (float), and strings (str).
- Integers: Whole numbers without decimals. For example:
1 |
age = 25 |
- Floats: Numbers with decimals. For example:
1 |
price = 19.99 |
- Strings: Sequences of characters enclosed in single or double quotes. For example:
1 |
name = "Alice" |
Declaring variables and assigning values
To declare a variable, simply choose a name and use the assignment operator (=) to give it a value.
1 2 |
city = "New York" population = 8500000 |
Dynamic typing: Python’s flexibility
Python is dynamically typed, meaning you don’t need to explicitly specify a variable’s data type. You can change a variable’s value and data type as needed:
1 2 |
x = 5 # x is an integer x = "hello" # now x is a string |
3. Performing Basic Operations
Now that we understand variables and data types, let’s dive into basic arithmetic operations in Python.
Arithmetic operations (+, -, *, /, %)
Python supports common arithmetic operations:
- Addition (+): Adds two numbers.
- Subtraction (–): Subtracts the second number from the first.
- Multiplication (*): Multiplies two numbers.
- Division (/): Divides the first number by the second.
- Modulus (%): Returns the remainder of division.
1 2 3 4 5 6 7 8 |
num1 = 10 num2 = 5 sum_result = num1 + num2 difference_result = num1 - num2 product_result = num1 * num2 quotient_result = num1 / num2 remainder_result = num1 % num2 |
Operator precedence: Getting the right result
When performing multiple operations in an expression, Python follows operator precedence. For example, multiplication and division take precedence over addition and subtraction.
1 |
result = 10 + 5 * 2 # Result is 20, not 30 |
Combining operations: Creating complex expressions
You can create complex expressions using parentheses to control the order of operations.
1 |
complex_result = (10 + 5) * 2 # Result is 30 |
4. Taking User Input
Building an interactive program involves taking input from users. Python provides a handy function called input() to achieve this.
Utilizing the input() function
The input() function prompts the user for input and returns the entered value as a string.
1 |
name = input("Please enter your name: ") |
Converting input to the desired data type
Often, you’ll want to perform operations on user input as numbers rather than strings. Use type conversion to achieve this.
1 2 |
age = input("Please enter your age: ") age_int = int(age) # Convert the string to an integer |
Building an interactive calculator
Now, let’s combine what we’ve learned to create an interactive calculator. We’ll take two numbers and an operator as input, then perform the calculation.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
num1 = float(input("Enter the first number: ")) num2 = float(input("Enter the second number: ")) operator = input("Enter the operator (+, -, *, /): ") if operator == "+": result = num1 + num2 elif operator == "-": result = num1 - num2 elif operator == "*": result = num1 * num2 elif operator == "/": result = num1 / num2 else: result = "Invalid operator" print("Result:", result) |
Try running the code and test out the calculator by entering two numbers and an operator. Experiment with different calculations!
5. Conditional Statements and Loops
Conditional statements and loops are essential tools in programming that enable you to make decisions and repeat tasks.
Making decisions with if statements
Conditional statements, often implemented using the if, elif (else if), and else keywords, allow your program to take different paths based on certain conditions.
1 2 3 4 5 6 7 8 |
age = int(input("Enter your age: ")) if age < 18: print("You are a minor.") elif age >= 18 and age < 65: print("You are an adult.") else: print("You are a senior citizen.") |
Performing repetitive tasks with loops
Loops enable you to execute a block of code multiple times. The two main types of loops in Python are the for loop and the while loop.
- For loop: Iterates over a sequence (like a list or range).
1 2 |
for i in range(5): print(i) |
- While loop: Repeats as long as a condition is true.
1 2 3 4 |
num = 0 while num < 5: print(num) num += 1 |
Enhancing the calculator with conditions
Let’s improve our calculator by adding validation and the ability to perform multiple calculations.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
while True: num1 = float(input("Enter the first number: ")) num2 = float(input("Enter the second number: ")) operator = input("Enter the operator (+, -, *, /): ") if operator == "+": result = num1 + num2 elif operator == "-": result = num1 - num2 elif operator == "*": result = num1 * num2 elif operator == "/": result = num1 / num2 else: print("Invalid operator") continue print("Result:", result) again = input("Do you want to perform another calculation? (yes/no): ") if again.lower() != "yes": break |
This enhanced calculator allows users to perform multiple calculations until they decide to stop.
6. Creating Functions
Functions are blocks of reusable code that can be called with different inputs. They help organize your code and make it more modular.
Understanding functions and their benefits
Functions in Python are defined using the def keyword, followed by the function name and parentheses containing any parameters.
1 2 |
def greet(name): print("Hello, " + name + "!") |
Benefits of using functions:
- Reusability: Write code once, use it many times.
- Modularity: Break down complex tasks into manageable chunks.
- Readability: Organize your code and make it more understandable.
Defining and calling functions
Once a function is defined, you can call it by using its name followed by parentheses.
1 2 3 4 5 |
def add(a, b): return a + b result = add(10, 5) # Call the add function print("Result:", result) |
Implementing functions in the calculator
Let’s enhance our calculator even further by incorporating functions.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
def add(a, b): return a + b def subtract(a, b): return a - b def multiply(a, b): return a * b def divide(a, b): if b != 0: return a / b else: return "Cannot divide by zero" while True: num1 = float(input("Enter the first number: ")) num2 = float(input("Enter the second number: ")) operator = input("Enter the operator (+, -, *, /): ") if operator == "+": result = add(num1, num2) elif operator == "-": result = subtract(num1, num2) elif operator == "*": result = multiply(num1, num2) elif operator == "/": result = divide(num1, num2) else: print("Invalid operator") continue print("Result:", result) again = input("Do you want to perform another calculation? (yes/no): ") if again.lower() != "yes": break |
7. Handling Errors
Error handling is crucial for creating robust programs. Python provides mechanisms to catch and manage errors gracefully.
Identifying and handling common errors
Common errors include division by zero (ZeroDivisionError), invalid input (ValueError), and more. Use try and except blocks to handle these errors.
1 2 3 4 5 6 7 8 |
try: num = int(input("Enter a number: ")) result = 10 / num print("Result:", result) except ZeroDivisionError: print("Cannot divide by zero") except ValueError: print("Invalid input. Please enter a valid number.") |
Using try-except for graceful error handling
By using try and except, your program can continue running even if an error occurs. This prevents crashes and improves user experience.
Ensuring your calculator is robust
Let’s integrate error handling into our calculator to make it more robust.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
while True: try: num1 = float(input("Enter the first number: ")) num2 = float(input("Enter the second number: ")) operator = input("Enter the operator (+, -, *, /): ") if operator == "+": result = add(num1, num2) elif operator == "-": result = subtract(num1, num2) elif operator == "*": result = multiply(num1, num2) elif operator == "/": result = divide(num1, num2) else: print("Invalid operator") continue print("Result:", result) except ValueError: print("Invalid input. Please enter valid numbers.") except ZeroDivisionError: print("Cannot divide by zero") again = input("Do you want to perform another calculation? (yes/no): ") if again.lower() != "yes": break |
8. Putting It All Together: Building Your Calculator
Congratulations on reaching this stage! We’ve covered a lot of ground in mastering Python basics. Now, let’s bring everything together and build your very own calculator with a simple user interface.
Designing the calculator’s user interface
For our calculator, we’ll create a user-friendly interface using the input() function to take user input.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
def add(a, b): return a + b def subtract(a, b): return a - b def multiply(a, b): return a * b def divide(a, b): if b != 0: return a / b else: return "Cannot divide by zero" print("Welcome to the Simple Calculator!") print("Available operators: +, -, *, /") while True: try: num1 = float(input("Enter the first number: ")) num2 = float(input("Enter the second number: ")) operator = input("Enter the operator: ") if operator == "+": result = add(num1, num2) elif operator == "-": result = subtract(num1, num2) elif operator == "*": result = multiply(num1, num2) elif operator == "/": result = divide(num1, num2) else: print("Invalid operator") continue print("Result:", result) except ValueError: print("Invalid input. Please enter valid numbers.") except ZeroDivisionError: print("Cannot divide by zero") again = input("Do you want to perform another calculation? (yes/no): ") if again.lower() != "yes": break |
Simple Calculator in Google Colab
Enhancing functionality through functions
By incorporating functions, error handling, and a user interface, we’ve built a simple yet effective calculator. This calculator can handle basic arithmetic operations, gracefully handle errors, and allow users to perform calculations in a user-friendly manner.
9. Frequently Asked Questions (FAQs)
What is Python mainly used for?
Python is a versatile programming language used for various purposes, including web development, data analysis, scientific computing, automation, artificial intelligence, and more.
How do I install Python on my computer?
To install Python, visit the official Python website, download the appropriate version for your operating system, and follow the installation instructions.
Can I write Python code in any text editor?
Yes, you can write Python code in any text editor. However, using integrated development environments (IDEs) like Visual Studio Code, PyCharm, or Jupyter Notebook can enhance your coding experience.
10. Conclusion
Congratulations on completing your journey through the fundamentals of Python programming by building a simple yet powerful calculator! You’ve explored a range of topics, from understanding variables and data types to creating functions and handling errors. As you reflect on your progress, remember that mastery comes with practice and continuous learning.
The power of practice and experimentation
Coding is a dynamic and creative process. The more you practice and experiment with Python, the more confident and capable you’ll become. As you dive deeper into the world of programming, consider taking on more complex projects that align with your interests. Each challenge you tackle will contribute to your growth as a programmer.
Continuing your Python journey
While this article provides a solid foundation, Python offers an extensive universe of possibilities to explore. As you advance, consider delving into topics like object-oriented programming, web development frameworks, data manipulation, and machine learning. Online resources, tutorials, and coding communities can be invaluable companions on your learning journey.
Remember, every programmer started as a beginner. Embrace the learning process, and don’t be afraid to ask questions, seek help, and learn from your mistakes. The road ahead is full of exciting opportunities, and Python is your trusty companion as you navigate the world of programming.
Thank you for joining us on this adventure into Python basics. Happy coding!
Online Compiler and Hands-On Practice
To solidify your understanding of the concepts covered in this article, we encourage you to try out the Python code snippets in an online compiler. This hands-on practice will help you gain confidence in writing and executing Python code.
Visit our Online Python Compiler or the Compiler given at the end of this blog post and input the code examples provided in each section. You can modify the code, experiment with different values, and observe the results. Remember, practice is key to mastering any skill, and programming is no exception.
Feel free to explore and create your own variations of the calculator, experiment with different functions, and even customize the user interface. The more you engage with the code, the better your understanding and skills will become.
Embrace the Journey
As you continue your Python programming journey, remember that every step you take is a valuable learning experience. From your first “Hello, Python!” program to building more complex applications, each achievement contributes to your growth as a developer. Don’t hesitate to revisit concepts, ask questions, and seek guidance from the programming community.
Python’s simplicity and versatility make it a fantastic language for both beginners and seasoned developers. So keep coding, keep learning, and keep pushing your boundaries. With dedication and practice, you’ll soon find yourself creating impressive applications and solving real-world problems using Python.
Thank you for joining us in this exploration of Python basics through the project of building a simple calculator. We hope you’ve gained valuable insights and are excited to embark on your coding journey. Happy coding!
Python Learning Resources
- Python.org’s Official Documentation – https://docs.python.org/ Python’s official documentation is a highly authoritative source. It provides in-depth information about the language, libraries, and coding practices. This is a go-to resource for both beginners and experienced developers.
- Coursera’s Python for Everybody Course – https://www.coursera.org/specializations/python Coursera hosts this popular course taught by Dr. Charles Severance. It covers Python programming from the ground up and is offered by the University of Michigan. The association with a reputable institution adds to its credibility.
- Real Python’s Tutorials and Articles – https://realpython.com/ Real Python is known for its high-quality tutorials and articles that cater to different skill levels. The platform is respected within the Python community for its accuracy and practical insights.
- Stack Overflow’s Python Tag – https://stackoverflow.com/questions/tagged/python Stack Overflow is a well-known platform for programming-related queries. Linking to the Python tag page can provide readers with access to a vast collection of real-world coding problems and solutions.
- Python Weekly Newsletter – https://www.pythonweekly.com/ The Python Weekly newsletter delivers curated content about Python programming, including articles, news, tutorials, and libraries. Subscribing to such newsletters is a common practice among developers looking for trustworthy updates.
Python projects and tools
- Free Python Compiler: Compile your Python code hassle-free with our online tool.
- Python Practice Ideas: Get inspired with 600+ programming ideas for honing your skills.
- Python Projects for Game Development: Dive into game development and unleash your creativity.
- Python Projects for IoT: Explore the exciting world of the Internet of Things through Python.
- Python for Artificial Intelligence: Discover how Python powers AI with 300+ projects.
- Comprehensive Python Project List: A one-stop collection of diverse Python projects.
- Python for Data Science: Harness Python’s potential for data analysis and visualization.
- Python for Web Development: Learn how Python is used to create dynamic web applications.
- Python Practice Platforms and Communities: Engage with fellow learners and practice your skills in real-world scenarios.
- Python Projects for All Levels: From beginner to advanced, explore projects tailored for every skill level.
- Python for Commerce Students: Discover how Python can empower students in the field of commerce.
1 thought on “Building a Simple Calculator: Free Python Course Online Module 1”